Float and double data types area a bad choice for embedded applications. At least in most applications, and can or should be avoided, even with hardware FPU support present.
But how can I be sure that no floating point operations are used?
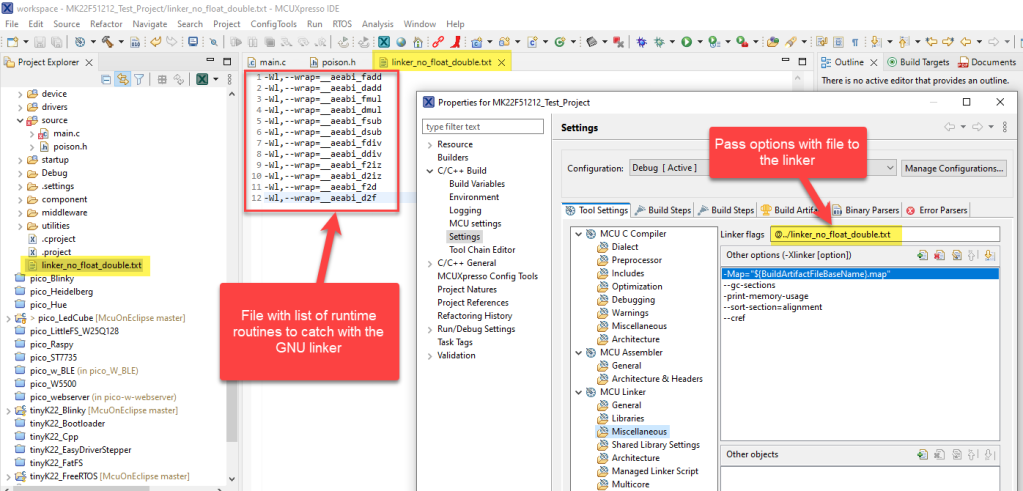
This article describes how to configure the GNU toolchain, so that no float or double operations are used, with the example of ARM Cortex-M. What I do? ‘Poisoning’ (!!!) the source code, force the gcc compiler to use software floating point operations and then catch them with the GNU linker :-).
Continue reading